In this document
Key classes
A toggle button allows the user to change a setting between two states.
You can add a basic toggle button to your layout with the ToggleButton
object. Android 4.0 (API level 14) introduces another kind of toggle button called a switch that
provides a slider control, which you can add with a Switch
object.
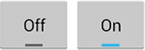
Toggle buttons

Switches (in Android 4.0+)
The ToggleButton
and Switch
controls are subclasses of CompoundButton
and function in the same manner, so
you can implement their behavior the same way.
Responding to Click Events
When the user selects a ToggleButton
and Switch
,
the object receives an on-click event.
To define the click event handler, add the android:onClick
attribute to the
<ToggleButton>
or <Switch>
element in your XML
layout. The value for this attribute must be the name of the method you want to call in response
to a click event. The Activity
hosting the layout must then implement the
corresponding method.
For example, here's a ToggleButton
with the android:onClick
attribute:
<ToggleButton android:id="@+id/togglebutton" android:layout_width="wrap_content" android:layout_height="wrap_content" android:textOn="Vibrate on" android:textOff="Vibrate off" android:onClick="onToggleClicked"/>
Within the Activity
that hosts this layout, the following method handles the
click event:
public void onToggleClicked(View view) { // Is the toggle on? boolean on = ((ToggleButton) view).isChecked(); if (on) { // Enable vibrate } else { // Disable vibrate } }
The method you declare in the android:onClick
attribute
must have a signature exactly as shown above. Specifically, the method must:
Tip: If you need to change the state
yourself,
use the setChecked(boolean)
or toggle()
method to change the state.
Using an OnCheckedChangeListener
You can also declare a click event handler pragmatically rather than in an XML layout. This
might be necessary if you instantiate the ToggleButton
or Switch
at runtime or you need to
declare the click behavior in a Fragment
subclass.
To declare the event handler programmatically, create an CompoundButton.OnCheckedChangeListener
object and assign it to the button by calling
setOnCheckedChangeListener(CompoundButton.OnCheckedChangeListener)
. For example:
ToggleButton toggle = (ToggleButton) findViewById(R.id.togglebutton); toggle.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() { public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) { if (isChecked) { // The toggle is enabled } else { // The toggle is disabled } } });