This lesson discusses how to create an implementation that mirrors newer APIs yet supports older devices.
Decide on a Substitute Solution
The most challenging task in using newer UI features in a backward-compatible way is deciding on and implementing an older (fallback) solution for older platform versions. In many cases, it's possible to fulfill the purpose of these newer UI components using older UI framework features. For example:
-
Action bars can be implemented using a horizontal
LinearLayout
containing image buttons, either as custom title bars or as views in your activity layout. Overflow actions can be presented under the device Menu button. -
Action bar tabs can be implemented using a horizontal
LinearLayout
containing buttons, or using theTabWidget
UI element. -
NumberPicker
andSwitch
widgets can be implemented usingSpinner
andToggleButton
widgets, respectively. -
ListPopupWindow
andPopupMenu
widgets can be implemented usingPopupWindow
widgets.
There generally isn't a one-size-fits-all solution for backporting newer UI components to older devices. Be mindful of the user experience: on older devices, users may not be familiar with newer design patterns and UI components. Give some thought as to how the same functionality can be delivered using familiar elements. In many cases this is less of a concern—if newer UI components are prominent in the application ecosystem (such as the action bar), or where the interaction model is extremely simple and intuitive (such as swipe views using a ViewPager
).
Implement Tabs Using Older APIs
To create an older implementation of action bar tabs, you can use a TabWidget
and TabHost
(although one can alternatively use horizontally laid-out Button
widgets). Implement this in classes called TabHelperEclair
and CompatTabEclair
, since this implementation uses APIs introduced no later than Android 2.0 (Eclair).
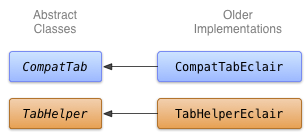
Figure 1. Class diagram for the Eclair implementation of tabs.
The CompatTabEclair
implementation stores tab properties such as the tab text and icon in instance variables, since there isn't an ActionBar.Tab
object available to handle this storage:
public class CompatTabEclair extends CompatTab {
// Store these properties in the instance,
// as there is no ActionBar.Tab object.
private CharSequence mText;
...
public CompatTab setText(int resId) {
// Our older implementation simply stores this
// information in the object instance.
mText = mActivity.getResources().getText(resId);
return this;
}
...
// Do the same for other properties (icon, callback, etc.)
}
The TabHelperEclair
implementation makes use of methods on the
TabHost
widget for creating TabHost.TabSpec
objects and tab indicators:
public class TabHelperEclair extends TabHelper {
private TabHost mTabHost;
...
protected void setUp() {
if (mTabHost == null) {
// Our activity layout for pre-Honeycomb devices
// must contain a TabHost.
mTabHost = (TabHost) mActivity.findViewById(
android.R.id.tabhost);
mTabHost.setup();
}
}
public void addTab(CompatTab tab) {
...
TabSpec spec = mTabHost
.newTabSpec(tag)
.setIndicator(tab.getText()); // And optional icon
...
mTabHost.addTab(spec);
}
// The other important method, newTab() is part of
// the base implementation.
}
You now have two implementations of CompatTab
and TabHelper
: one that works on devices running Android 3.0 or later and uses new APIs, and another that works on devices running Android 2.0 or later and uses older APIs. The next lesson discusses using these implementations in your application.