This lesson teaches you to
You should also read
Implementing an effective and user friendly share action in your ActionBar
is made even easier with the introduction of ActionProvider
in Android 4.0
(API Level 14). An ActionProvider
, once attached to a menu item in the action
bar, handles both the appearance and behavior of that item. In the case of ShareActionProvider
, you provide a share intent and it does the rest.
Note: ShareActionProvider
is available
starting with API Level 14 and higher.
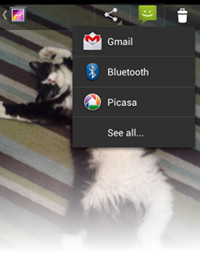
Figure 1. The ShareActionProvider
in the Gallery app.
Update Menu Declarations
To get started with ShareActionProviders
, define the android:actionProviderClass
attribute for the corresponding <item>
in your menu resource file:
<menu xmlns:android="http://schemas.android.com/apk/res/android">
<item android:id="@+id/menu_item_share"
android:showAsAction="ifRoom"
android:title="Share"
android:actionProviderClass="android.widget.ShareActionProvider" />
...
</menu>
This delegates responsibility for the item's appearance and function to
ShareActionProvider
. However, you will need to tell the provider what you
would like to share.
Set the Share Intent
In order for ShareActionProvider
to function, you must provide it a share
intent. This share intent should be the same as described in the Sending Content to Other Apps
lesson, with action ACTION_SEND
and additional data set via extras
like EXTRA_TEXT
and EXTRA_STREAM
. To
assign a share intent, first find the corresponding MenuItem
while inflating
your menu resource in your Activity
or Fragment
. Next, call
MenuItem.getActionProvider()
to retreive an
instance of ShareActionProvider
. Use setShareIntent()
to
update the share intent associated with that action item. Here's an example:
private ShareActionProvider mShareActionProvider;
...
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate menu resource file.
getMenuInflater().inflate(R.menu.share_menu, menu);
// Locate MenuItem with ShareActionProvider
MenuItem item = menu.findItem(R.id.menu_item_share);
// Fetch and store ShareActionProvider
mShareActionProvider = (ShareActionProvider) item.getActionProvider();
// Return true to display menu
return true;
}
// Call to update the share intent
private void setShareIntent(Intent shareIntent) {
if (mShareActionProvider != null) {
mShareActionProvider.setShareIntent(shareIntent);
}
}
You may only need to set the share intent once during the creation of your menus, or you may want to set it and then update it as the UI changes. For example, when you view photos full screen in the Gallery app, the sharing intent changes as you flip between photos.
For further discussion about the ShareActionProvider
object, see the Action Bar guide.