Making objects move according to a preset program like the rotating triangle is useful for
getting some attention, but what if you want to have users interact with your OpenGL ES graphics?
The key to making your OpenGL ES application touch interactive is expanding your implementation of
GLSurfaceView
to override the onTouchEvent()
to listen for touch events.
This lesson shows you how to listen for touch events to let users rotate an OpenGL ES object.
Setup a Touch Listener
In order to make your OpenGL ES application respond to touch events, you must implement the
onTouchEvent()
method in your
GLSurfaceView
class. The example implementation below shows how to listen for
MotionEvent.ACTION_MOVE
events and translate them to
an angle of rotation for a shape.
@Override public boolean onTouchEvent(MotionEvent e) { // MotionEvent reports input details from the touch screen // and other input controls. In this case, you are only // interested in events where the touch position changed. float x = e.getX(); float y = e.getY(); switch (e.getAction()) { case MotionEvent.ACTION_MOVE: float dx = x - mPreviousX; float dy = y - mPreviousY; // reverse direction of rotation above the mid-line if (y > getHeight() / 2) { dx = dx * -1 ; } // reverse direction of rotation to left of the mid-line if (x < getWidth() / 2) { dy = dy * -1 ; } mRenderer.mAngle += (dx + dy) * TOUCH_SCALE_FACTOR; // = 180.0f / 320 requestRender(); } mPreviousX = x; mPreviousY = y; return true; }
Notice that after calculating the rotation angle, this method calls requestRender()
to tell the
renderer that it is time to render the frame. This approach is the most efficient in this example
because the frame does not need to be redrawn unless there is a change in the rotation. However, it
does not have any impact on efficiency unless you also request that the renderer only redraw when
the data changes using the setRenderMode()
method, so make sure this line is uncommented in the renderer:
public MyGLSurfaceView(Context context) { ... // Render the view only when there is a change in the drawing data setRenderMode(GLSurfaceView.RENDERMODE_WHEN_DIRTY); }
Expose the Rotation Angle
The example code above requires that you expose the rotation angle through your renderer by
adding a public member. Since the renderer code is running on a separate thread from the main user
interface thread of your application, you must declare this public variable as volatile
.
Here is the code to do that:
public class MyGLRenderer implements GLSurfaceView.Renderer { ... public volatile float mAngle;
Apply Rotation
To apply the rotation generated by touch input, comment out the code that generates an angle and
add mAngle
, which contains the touch input generated angle:
public void onDrawFrame(GL10 gl) { ... // Create a rotation for the triangle // long time = SystemClock.uptimeMillis() % 4000L; // float angle = 0.090f * ((int) time); Matrix.setRotateM(mRotationMatrix, 0, mAngle, 0, 0, -1.0f); // Combine the rotation matrix with the projection and camera view Matrix.multiplyMM(mMVPMatrix, 0, mRotationMatrix, 0, mMVPMatrix, 0); // Draw triangle mTriangle.draw(mMVPMatrix); }
When you have completed the steps described above, run the program and drag your finger over the screen to rotate the triangle:
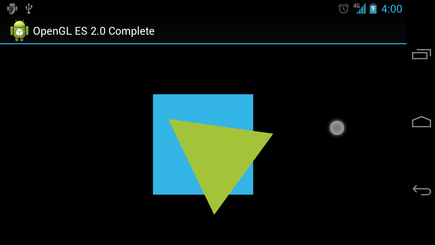
Figure 1. Triangle being rotated with touch input (circle shows touch location).