Properly stopping and restarting your activity is an important process in the activity lifecycle that ensures your users perceive that your app is always alive and doesn't lose their progress. There are a few of key scenarios in which your activity is stopped and restarted:
- The user opens the Recent Apps window and switches from your app to another app. The activity in your app that's currently in the foreground is stopped. If the user returns to your app from the Home screen launcher icon or the Recent Apps window, the activity restarts.
- The user performs an action in your app that starts a new activity. The current activity is stopped when the second activity is created. If the user then presses the Back button, the first activity is restarted.
- The user receives a phone call while using your app on his or her phone.
The Activity
class provides two lifecycle methods, onStop()
and onRestart()
, which allow you to
specifically handle how your activity handles being stopped and restarted. Unlike the paused state,
which identifies a partial UI obstruction, the stopped state guarantees that the UI is no longer
visible and the user's focus is in a separate activity (or an entirely separate app).
Note: Because the system retains your Activity
instance in system memory when it is stopped, it's possible that you don't need to implement the
onStop()
and onRestart()
(or even onStart()
methods at all. For most activities that are relatively simple, the
activity will stop and restart just fine and you might only need to use onPause()
to pause ongoing actions and disconnect from system resources.
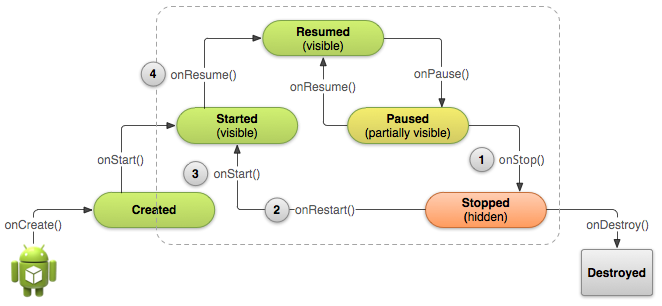
Figure 1. When the user leaves your activity, the system
calls onStop()
to stop the activity (1). If the user returns
while the activity is stopped, the system calls onRestart()
(2), quickly followed by onStart()
(3) and onResume()
(4). Notice that no matter what scenario causes the activity to
stop, the system always calls onPause()
before calling onStop()
.
Stop Your Activity
When your activity receives a call to the onStop()
method, it's no
longer visible and should release almost all resources that aren't needed while the user is not
using it. Once your activity is stopped, the system might destroy the instance if it needs to
recover system memory. In extreme cases, the system might simply kill your app process without
calling the activity's final onDestroy()
callback, so it's important
you use onStop()
to release resources that might leak memory.
Although the onPause()
method is called before
onStop()
, you should use onStop()
to perform larger, more CPU intensive shut-down operations, such as writing information to a
database.
For example, here's an implementation of onStop()
that
saves the contents of a draft note to persistent storage:
@Override protected void onStop() { super.onStop(); // Always call the superclass method first // Save the note's current draft, because the activity is stopping // and we want to be sure the current note progress isn't lost. ContentValues values = new ContentValues(); values.put(NotePad.Notes.COLUMN_NAME_NOTE, getCurrentNoteText()); values.put(NotePad.Notes.COLUMN_NAME_TITLE, getCurrentNoteTitle()); getContentResolver().update( mUri, // The URI for the note to update. values, // The map of column names and new values to apply to them. null, // No SELECT criteria are used. null // No WHERE columns are used. ); }
When your activity is stopped, the Activity
object is kept resident in memory
and is recalled when the activity resumes. You don’t need to re-initialize components that were
created during any of the callback methods leading up to the Resumed state. The system also
keeps track of the current state for each View
in the layout, so if the user
entered text into an EditText
widget, that content is retained so you don't
need to save and restore it.
Note: Even if the system destroys your activity while it's stopped,
it still retains the state of the View
objects (such as text in an EditText
) in a Bundle
(a blob of key-value pairs) and restores
them if the user navigates back to the same instance of the activity (the next lesson talks more about using a Bundle
to save
other state data in case your activity is destroyed and recreated).
Start/Restart Your Activity
When your activity comes back to the foreground from the stopped state, it receives a call to
onRestart()
. The system also calls the onStart()
method, which happens every time your activity becomes visible
(whether being restarted or created for the first time). The onRestart()
method, however, is called only when the activity resumes from the
stopped state, so you can use it to perform special restoration work that might be necessary only if
the activity was previously stopped, but not destroyed.
It's uncommon that an app needs to use onRestart()
to restore
the activity's state, so there aren't any guidelines for this method that apply to
the general population of apps. However, because your onStop()
method should essentially clean up all your activity's resources, you'll need to re-instantiate them
when the activity restarts. Yet, you also need to instantiate them when your activity is created
for the first time (when there's no existing instance of the activity). For this reason, you
should usually use the onStart()
callback method as the counterpart
to the onStop()
method, because the system calls onStart()
both when it creates your activity and when it restarts the
activity from the stopped state.
For example, because the user might have been away from your app for a long time before
coming back it, the onStart()
method is a good place to verify that
required system features are enabled:
@Override protected void onStart() { super.onStart(); // Always call the superclass method first // The activity is either being restarted or started for the first time // so this is where we should make sure that GPS is enabled LocationManager locationManager = (LocationManager) getSystemService(Context.LOCATION_SERVICE); boolean gpsEnabled = locationManager.isProviderEnabled(LocationManager.GPS_PROVIDER); if (!gpsEnabled) { // Create a dialog here that requests the user to enable GPS, and use an intent // with the android.provider.Settings.ACTION_LOCATION_SOURCE_SETTINGS action // to take the user to the Settings screen to enable GPS when they click "OK" } } @Override protected void onRestart() { super.onRestart(); // Always call the superclass method first // Activity being restarted from stopped state }
When the system destroys your activity, it calls the onDestroy()
method for your Activity
. Because you should generally have released most of
your resources with onStop()
, by the time you receive a call to onDestroy()
, there's not much that most apps need to do. This method is your
last chance to clean out resources that could lead to a memory leak, so you should be sure that
additional threads are destroyed and other long-running actions like method tracing are also
stopped.