This lesson teaches you to
- Understand the Lifecycle Callbacks
- Specify Your App's Launcher Activity
- Create a New Instance
- Destroy the Activity
You should also read
Try it out
ActivityLifecycle.zip
Unlike other programming paradigms in which apps are launched with a main()
method, the
Android system initiates code in an Activity
instance by invoking specific
callback methods that correspond to specific stages of its
lifecycle. There is a sequence of callback methods that start up an activity and a sequence of
callback methods that tear down an activity.
This lesson provides an overview of the most important lifecycle methods and shows you how to handle the first lifecycle callback that creates a new instance of your activity.
Understand the Lifecycle Callbacks
During the life of an activity, the system calls a core set of lifecycle methods in a sequence similar to a step pyramid. That is, each stage of the activity lifecycle is a separate step on the pyramid. As the system creates a new activity instance, each callback method moves the activity state one step toward the top. The top of the pyramid is the point at which the activity is running in the foreground and the user can interact with it.
As the user begins to leave the activity, the system calls other methods that move the activity state back down the pyramid in order to dismantle the activity. In some cases, the activity will move only part way down the pyramid and wait (such as when the user switches to another app), from which point the activity can move back to the top (if the user returns to the activity) and resume where the user left off.
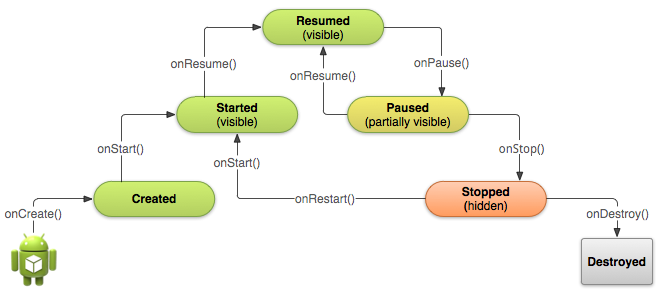
Figure 1. A simplified illustration of the Activity lifecycle, expressed as a step pyramid. This shows how, for every callback used to take the activity a step toward the Resumed state at the top, there's a callback method that takes the activity a step down. The activity can also return to the resumed state from the Paused and Stopped state.
Depending on the complexity of your activity, you probably don't need to implement all the lifecycle methods. However, it's important that you understand each one and implement those that ensure your app behaves the way users expect. Implementing your activity lifecycle methods properly ensures your app behaves well in several ways, including that it:
- Does not crash if the user receives a phone call or switches to another app while using your app.
- Does not consume valuable system resources when the user is not actively using it.
- Does not lose the user's progress if they leave your app and return to it at a later time.
- Does not crash or lose the user's progress when the screen rotates between landscape and portrait orientation.
As you'll learn in the following lessons, there are several situations in which an activity transitions between different states that are illustrated in figure 1. However, only three of these states can be static. That is, the activity can exist in one of only three states for an extended period of time:
- Resumed
- In this state, the activity is in the foreground and the user can interact with it. (Also sometimes referred to as the "running" state.)
- Paused
- In this state, the activity is partially obscured by another activity—the other activity that's in the foreground is semi-transparent or doesn't cover the entire screen. The paused activity does not receive user input and cannot execute any code.
- Stopped
- In this state, the activity is completely hidden and not visible to the user; it is considered to be in the background. While stopped, the activity instance and all its state information such as member variables is retained, but it cannot execute any code.
The other states (Created and Started) are transient and the system quickly moves from them to
the next state by calling the next lifecycle callback method. That is, after the system calls
onCreate()
, it quickly calls onStart()
, which is quickly followed by onResume()
.
That's it for the basic activity lifecycle. Now you'll start learning about some of the specific lifecycle behaviors.
Specify Your App's Launcher Activity
When the user selects your app icon from the Home screen, the system calls the onCreate()
method for the Activity
in your app
that you've declared to be the "launcher" (or "main") activity. This is the activity that serves as
the main entry point to your app's user interface.
You can define which activity to use as the main activity in the Android manifest file, AndroidManifest.xml
, which is
at the root of your project directory.
The main activity for your app must be declared in the manifest with an <intent-filter>
that includes the MAIN
action and
LAUNCHER
category. For example:
<activity android:name=".MainActivity" android:label="@string/app_name"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity>
Note: When you create a new Android project with the Android SDK
tools, the default project files include an Activity
class that's declared in
the manifest with this filter.
If either the MAIN
action or
LAUNCHER
category are not declared for one of your
activities, then your app icon will not appear in the Home screen's list of apps.
Create a New Instance
Most apps include several different activities that allow the user to perform different actions.
Whether an activity is the main activity that's created when the user clicks your app icon or a
different activity that your app starts in response to a user action, the system creates
every new instance of Activity
by calling its onCreate()
method.
You must implement the onCreate()
method to perform basic
application startup logic that should happen only once for the entire life of the activity. For
example, your implementation of onCreate()
should define the
user interface and possibly instantiate some class-scope variables.
For example, the following example of the onCreate()
method shows some code that performs some fundamental setup for the activity, such as
declaring the user interface (defined in an XML layout file), defining member variables,
and configuring some of the UI.
TextView mTextView; // Member variable for text view in the layout @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); // Set the user interface layout for this Activity // The layout file is defined in the project res/layout/main_activity.xml file setContentView(R.layout.main_activity); // Initialize member TextView so we can manipulate it later mTextView = (TextView) findViewById(R.id.text_message); // Make sure we're running on Honeycomb or higher to use ActionBar APIs if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.HONEYCOMB) { // For the main activity, make sure the app icon in the action bar // does not behave as a button ActionBar actionBar = getActionBar(); actionBar.setHomeButtonEnabled(false); } }
Caution: Using the SDK_INT
to
prevent older system's from executing new APIs works in this way on Android 2.0 (API level
5) and higher only. Older versions will encounter a runtime exception.
Once the onCreate()
finishes execution, the system
calls the onStart()
and onResume()
methods
in quick succession. Your activity never resides in the Created or Started states. Technically, the
activity becomes visible to the user when onStart()
is called, but
onResume()
quickly follows and the activity remains in the Resumed
state until something occurs to change that, such as when a phone call is received, the user
navigates to another activity, or the device screen turns off.
In the other lessons that follow, you'll see how the other start up methods, onStart()
and onResume()
, are useful during your
activity's lifecycle when used to resume the activity from the Paused or Stopped states.
Note: The onCreate()
method includes a parameter called savedInstanceState
that's discussed in the
latter lesson about Recreating an Activity.
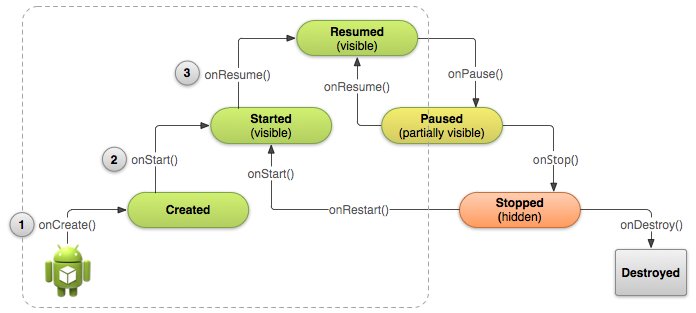
Figure 2. Another illustration of the activity lifecycle
structure with an emphasis on the three main callbacks that the system calls in sequence when
creating a new instance of the activity: onCreate()
, onStart()
, and onResume()
. Once this sequence of
callbacks complete, the activity reaches the Resumed state where users can interact with the
activity until they switch to a different activity.
Destroy the Activity
While the activity's first lifecycle callback is onCreate()
, its very last callback is onDestroy()
. The system calls
this method on your activity as the final
signal that your activity instance is being completely removed from the system memory.
Most apps don't need to implement this method because local class references are destroyed
with the activity and your activity should perform most cleanup during onPause()
and onStop()
. However, if your
activity includes background threads that you created during onCreate()
or other long-running resources that could
potentially leak memory if not properly closed, you should kill them during onDestroy()
.
@Override public void onDestroy() { super.onDestroy(); // Always call the superclass // Stop method tracing that the activity started during onCreate() android.os.Debug.stopMethodTracing(); }
Note: The system calls onDestroy()
after it has already called onPause()
and onStop()
in all situations except one: when you call finish()
from within the onCreate()
method. In some cases, such as when your activity operates as a temporary decision maker to
launch another activity, you might call finish()
from within onCreate()
to destroy the activity. In this case, the system
immediately calls onDestroy()
without calling any of the other
lifecycle methods.