During normal app use, the foreground activity is sometimes obstructed by other visual components that cause the activity to pause. For example, when a semi-transparent activity opens (such as one in the style of a dialog), the previous activity pauses. As long as the activity is still partially visible but currently not the activity in focus, it remains paused.
However, once the activity is fully-obstructed and not visible, it stops (which is discussed in the next lesson).
As your activity enters the paused state, the system calls the onPause()
method on your Activity
, which allows
you to stop ongoing actions that should not continue while paused (such as a video) or persist
any information that should be permanently saved in case the user continues to leave your app. If
the user returns to your activity from the paused state, the system resumes it and calls the
onResume()
method.
Note: When your activity receives a call to onPause()
, it may be an indication that the activity will be paused for a
moment and the user may return focus to your activity. However, it's usually the first indication
that the user is leaving your activity.
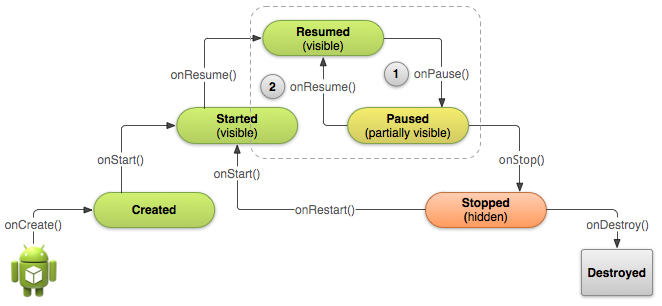
Figure 1. When a semi-transparent activity obscures
your activity, the system calls onPause()
and the activity
waits in the Paused state (1). If the user returns to the activity while it's still paused, the
system calls onResume()
(2).
Pause Your Activity
When the system calls onPause()
for your activity, it
technically means your activity is still partially visible, but most often is an indication that
the user is leaving the activity and it will soon enter the Stopped state. You should usually use
the onPause()
callback to:
- Stop animations or other ongoing actions that could consume CPU.
- Commit unsaved changes, but only if users expect such changes to be permanently saved when they leave (such as a draft email).
- Release system resources, such as broadcast receivers, handles to sensors (like GPS), or any resources that may affect battery life while your activity is paused and the user does not need them.
For example, if your application uses the Camera
, the
onPause()
method is a good place to release it.
@Override public void onPause() { super.onPause(); // Always call the superclass method first // Release the Camera because we don't need it when paused // and other activities might need to use it. if (mCamera != null) { mCamera.release() mCamera = null; } }
Generally, you should not use onPause()
to store
user changes (such as personal information entered into a form) to permanent storage. The only time
you should persist user changes to permanent storage within onPause()
is when you're certain users expect the changes to be auto-saved (such as when drafting an email).
However, you should avoid performing CPU-intensive work during onPause()
, such as writing to a database, because it can slow the visible
transition to the next activity (you should instead perform heavy-load shutdown operations during
onStop()
).
You should keep the amount of operations done in the onPause()
method relatively simple in order to allow for a speedy transition to the user's next
destination if your activity is actually being stopped.
Note: When your activity is paused, the Activity
instance is kept resident in memory and is recalled when the activity resumes.
You don’t need to re-initialize components that were created during any of the callback methods
leading up to the Resumed state.
Resume Your Activity
When the user resumes your activity from the Paused state, the system calls the onResume()
method.
Be aware that the system calls this method every time your activity comes into the foreground,
including when it's created for the first time. As such, you should implement onResume()
to initialize components that you release during onPause()
and perform any other initializations that must occur each time the
activity enters the Resumed state (such as begin animations and initialize components only used
while the activity has user focus).
The following example of onResume()
is the counterpart to
the onPause()
example above, so it initializes the camera that's
released when the activity pauses.
@Override public void onResume() { super.onResume(); // Always call the superclass method first // Get the Camera instance as the activity achieves full user focus if (mCamera == null) { initializeCamera(); // Local method to handle camera init } }